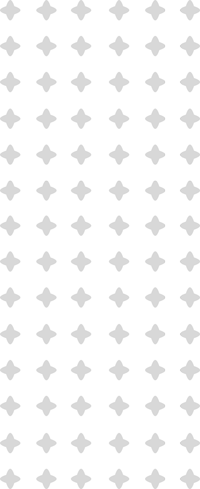
Courses Conducted
By M. M. Rahman
Professor, Dept. of Computer Science and Engineering, Jatiya Kabi Kazi Nazrul Islam University, Bangladesh.
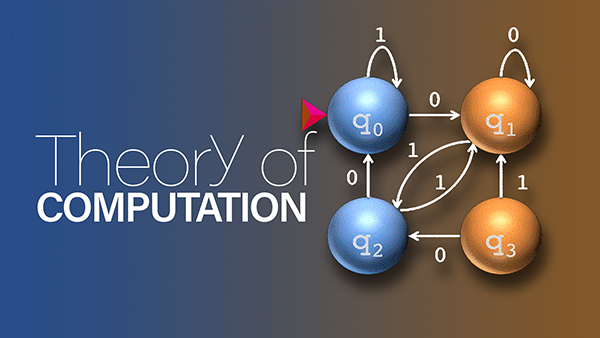
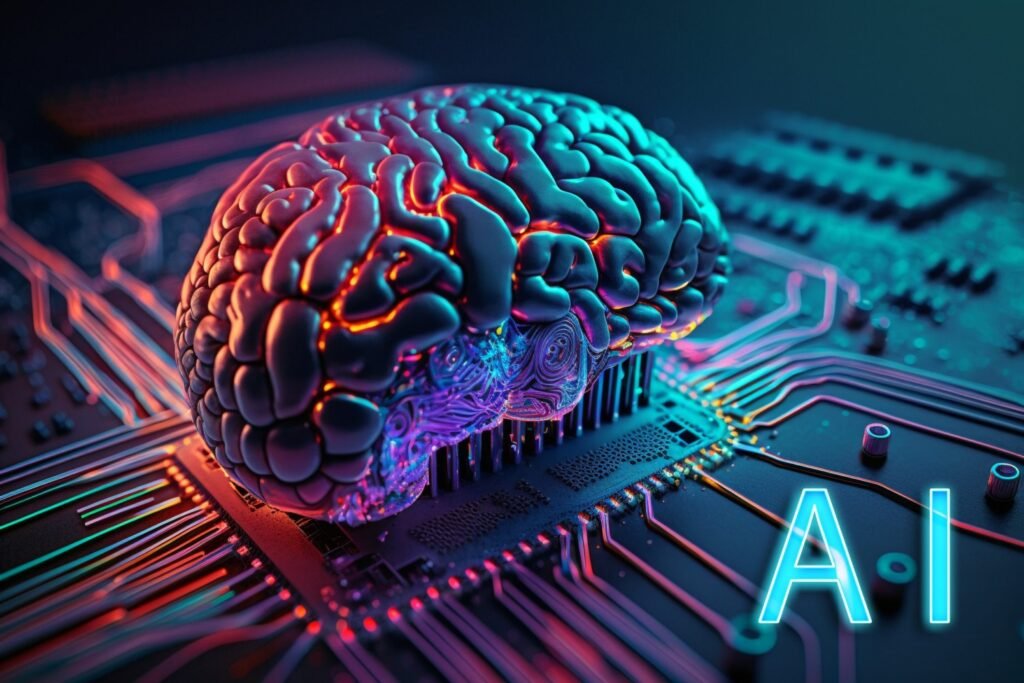
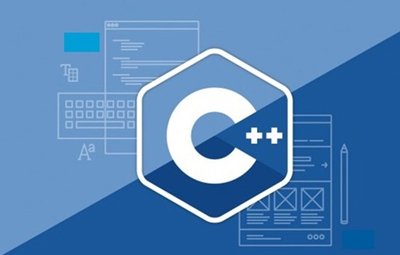
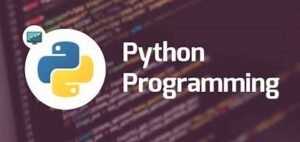
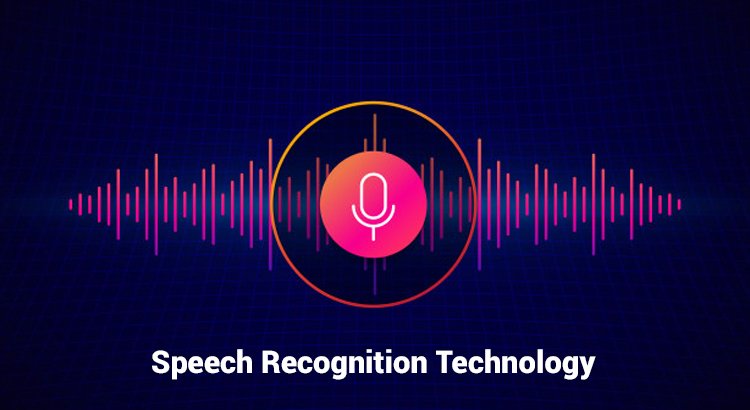
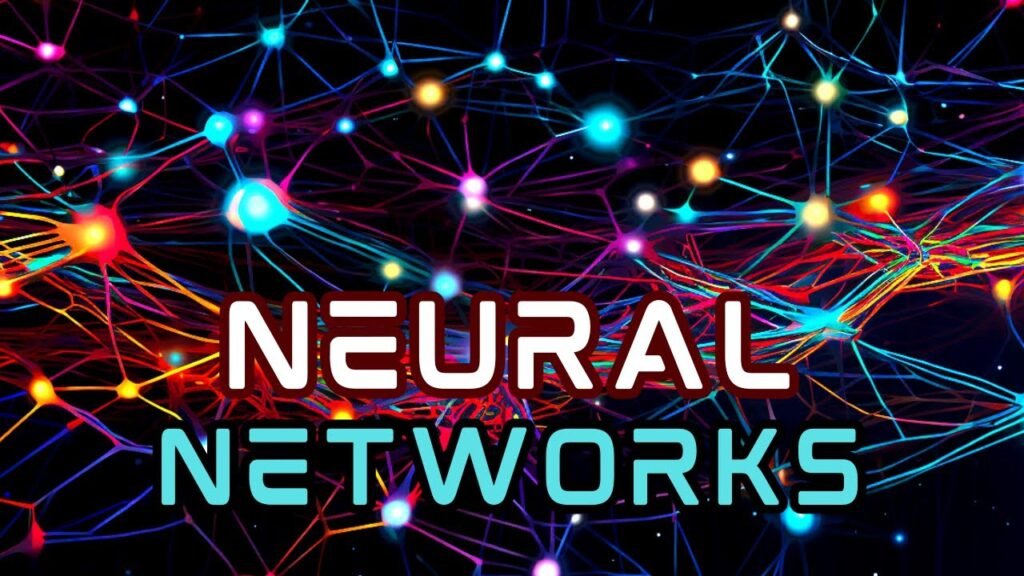
Structured Programming
This is an introductory course and covers the key features of the C language and its usage. This course is aimed at advancing concepts of programming and software code organization within the framework of structural and procedural programming paradigms. This course involves a lab component which is designed to give the student hands-on experience with the concepts. Students will be able to develop logic which will help them to create programs and applications in C. Also, by learning the basic programming constructs they can easily switch over to any other language in the future.
Course Contents
- About This course
- About Class and Course Evaluation
- The Grading System
- Course Prerequisites and Dependencies
- Course Objectives
- Course Contents
- Overall Teaching Goals
- Learning Outcomes
- Text Books and References
- The C Programming Language
- History of the C Language
- Features of C Language
- Preparing to C Program
- The C Development Cycle
- Your First C Program
- How C Programming Language Works?
- C Environment
- Key Uses and Applications
- Why Learn C Language?
- Sample C Programs
- Conditional Control Structures
- Decision making and looping (Iteration) statements:
- for loop
- while loop
- do…while loop
Lectures: SP#10
- Top-Down Modular Programming
- Functions in C
- Why do we need function?
- Function Types: User-defined and Library
- Elements of User-defined Function
- Function Definition
- Function Declaration
- Function Calls
- Category of Functions
- Parameters passing to Functions
- Main Function
- Library Functions
- Nesting of Functions
- Recursion
- What is Pointer in C?
- Memory Organization of Pointers
- Accessing the Address of a Variable
- Declaration of Pointer Variables
- Initialization of Pointer Variables
- Accessing a Variable Through Its Pointer
- Pointer Expressions
- Pointers and Arrays, and Array of Pointers
- Pointers and Strings
- Pointers and Functions
- Pointers and Structures
- Introduction
- Why do we need File Handling in C?
- Types of Files in C
- C File Operations
- File Pointer in C
- Functions for File Handling
- Defining and Opening a File
- Closing a File
- I/O Operations on Files
- Reading from a File
- Writing to a File
- Error Handling during File Operations
Text & Reference Books:
- Programming & Software Development with AI and Machine Learning Concepts, By M. M. Rahman
- Programming in ANSI C, By E. Balagurusamy
- Programming in C, By Stephen G. Kochan
- C: The Complete Reference, By Herbert Schildt
Online Course Materials:
- C Tutorial – Tutorialspoint.com
- C Programming Language Tutorial – javaTpoint.com
- C Programming Language Tutorial – geeksforgeeks.org
Online C Compiler:
Software (Code:: Blocks):
Programming with C++
"Programming with C++" is a foundational course designed to teach the fundamentals of the C++ programming language. This course is often part of computer science or engineering curricula and is suitable for beginners who are new to programming as well as those who have some prior experience with other languages. Key Topics Covered: Introduction to C++, Data Types and Variables, Control Structures, Functions, Arrays and Strings, Object-Oriented Programming (OOP) Concepts, Operator Overloading, File Handling.
Course Contents
- About This Course
- Course Outcomes
- About Class and Course Evaluation
- The Grading System
- Course Contents
- Course Objectives
- Course Prerequisites
- Text Books and References
- Computer Programming
- Software Development Stages
- Programming Languages
- Object-Oriented Programming (OOP)
- History of C++ Language
- Features of C++ Language
- C++ Program Structure
- C++ Tokens
- Simple C++ Programs
- C++ Identifiers
- C++ Keywords
- C++ Variables
- Types of C++ Variables
- Scope of Variables in C++
- Constants in C++
- C++ Data Types
- C++ Data Type Modifiers
- Type Conversion in C++
- Literals In C++
- C++ Operators
- C++ Input
- C++ Output
- Programming & Software Development with AI and Machine Learning Concepts, By M. M. Rahman
- Object Oriented programming with C++, By E. Balagurusamy
- The C++ Programming Language, By Bjarne Stroustrup
- The Complete Reference C++, By Herbert Schildt
- A Complete Guide to Programming in C++, By Ulla Kirch-Prinz
Theory of Computation
The "Theory of Computation" is a branch of computer science and mathematics that focuses on determining problems that can be solved mechanically, using an algorithm or a set of programming rules. It is also concerned with the efficiency at which the algorithm can perform the solution. Content of the course: Automata and Formal Languages, Computability, and Complexity Theory.
Course Contents
- About the course
- Purpose and Motivation
- What is the “Theory of Computation”?
- Why Study Theory of computation
- Course Contents
- Learning Outcomes
- List of Reference Books
- What is the “Theory of Computation”?
- History: Theory of Computation
- Branches of the Theory of Computation
- Automata Theory, and Formal Language
- Overview of Finite Automata, Context-free Grammars, Pushdown Automata, and Turing Machines
- Computability Theory, Complexity Theory, and Models of Computation
- Applications of Theory of computation
- Task and Problem
- Problem Representations
- Types of Problems
- Definitions, Theorems, Proofs
- Proof Techniques
- Direct proof technique
- Proof by construction
- Proof by contradiction
- Proof by counter example
- Proof by induction
- Proof by using pigeonhole principle
- Proof technique for if and only if statements
- Finite State Machine
- Types of Finite Automata
- Transition Function, Diagram and Table
- DFA, NFA, e-NFA with Definitions and Examples
- Extended Transition Function
- The Equivalence of NFA’s with and without e-moves
- Conversion of e-NFA into NFA (without e)
- Two-way FA
- FA with Output: Moore machine, Mealy machine, Equivalence
- Applications of FA
- The Equivalence of DFA’s and NFA’s
- Regular Expressions
- Regular Languages
- Operation on Regular Languages
- Extensions of Regular Expressions
- Regular Sets and Properties of Regular Sets
- Identities Related to Regular Expressions
- Conversion of Finite Automata into Regular Expressions
- Conversion of Regular Expressions into Finite Automata
- Pumping Lemma for Regular Languages
- Closure Properties of Regular Languages
- Relationship with other Computation Models
- Introduction to Context-Free Grammar (CFG)
- Formal Definition of Context-Free Grammar (CFG)
- Example of Context-Free Grammars
- Parse Trees
- Capabilities of CFG
- Relationship with other Computation Models
- Types of Context-Free Grammars
- Derivations Using Grammars
- Removal of Ambiguity
- Removal of Left Recursion
- Left Factoring
- Simplification of CFG
- Chomsky Normal Form (CNF)
- Theory of Computation, By Rajesh K. Shukla
- Introduction to the Theory of Computation, By Michael Sipser
- Introduction to Languages and The Theory of Computation, By John C Martin
- An Introduction to Formal Languages and Automata, By Peter Linz
- Introduction to Automata Theory, Languages, and Computation, By John E. Hopcroft and Jeffery D. Ullman
- Fundamentals of the Theory of Computation, By Raymond Green and H. James Hoover
Basic Programming with Python
The objectives of a Basic Programming with Python course are to provide learners with foundational knowledge and skills in programming using the Python programming language. A basic outline for a course on programming with Python includes Introduction to Python, Variables and Data Types, Operators and Expressions, Control Structures, Data Structures, Functions, Modules and Packages, File Handling, and Introduction to Object-Oriented Programming (OOP). This course aims to empower learners with essential programming skills and knowledge using Python, enabling them to solve problems, write efficient code, and pursue further studies or careers in computer science and related fields.
Course Contents
- Computer Programming
- Problem Statement
- Requirements Gathering
- Design
- Coding
- Testing
- Documentation
- Maintenance
- Programming Languages
- Programming (or Development) Tools
- Conditional Statements
- if Statement
- if…else Statement
- if…elif Statement
- Nested Conditionals
- Conditional Operator (Conditional Expression)
- Match-Case Statement
- Iteration (Loop) Statements
- for Loop
- for…else Loop
- while Loop
- while…else Loop
- Using else Statement with Loops
- Nested Loops
- Loop Control Statements
- Break Statement
- Continue Statement
- Pass Statement
- Data Structures
- List
- Tuple
- Set
- Dictionary
- String
- Arrays
Lecture 8
Lecture 9
Lecture 10
- Procedural Oriented Approach
- OOP Concepts
- Class and Object
- Methods in Python
- Program Examples Using Class, Object and Method
- Encapsulation in Python
- Static Variable and Static Method
- Constructor in Python
- Instance Variable and Instance Method
- Inheritance in Python
- Online IDE: Online Python Compiler (Interpreter): Write and run Python code using online compiler (interpreter). We can use Python Shell like IDLE, and take inputs from the user in the Python compiler.
- IDLE (Integrated Development and Learning Environment): IDLE is a default editor that accompanies Python. This IDE is suitable for beginner-level developers. The IDLE tool can be used on Mac OS, Windows, and Linux.
- Read More from: https://docs.python.org/3/library/idle.html
- PyCharm: PyCharm is a widely used Python IDE created by JetBrains. This IDE is suitable for professional developers and facilitates the development of large Python projects.How to install and set up PyCharm:
- Python in Visual Studio Code: Visual Studio Code is an open-source (and free) IDE created by Microsoft. It finds great use in Python development. VS Code is lightweight and comes with powerful features that only some of the paid IDEs offer.
- Jupyter Notebook: Jupyter is widely used in the field of data science. It is easy to use, interactive and allows live code sharing and visualization.
-
- Read More and Download Notebook: https://jupyter.org
-
Artificial Intelligence
Artificial Intelligence (AI) is a core course in Computer Science. The goal is to acquire knowledge of intelligent systems and agents, formalization of knowledge, reasoning with and without uncertainty, machine learning, and applications at a basic level.
Course Contents
- What is artificial intelligence (AI)?
- How does AI work?
- Types of AI
- What are examples of AI technology and how is it used today?
- Components of AI
- Objectives of this Course
- Course Contents
- Recommended Books
- Introduction to AI
- Major goals of AI
- Some definitions of AI
- History of AI
- The Foundations of AI
- How to work the human brain?
- Sub-fields of AI
- The State of the Art
- Components of AI System
- How does Artificial Intelligence work?
- Fundamental Techniques of AI
- Types of AI
- AI in Everyday Life
- What is an (Intelligent) Agent?
- Structure of an Intelligent Agent
- Major Features of Agents
- PAGE Properties
- A Simple Agent Example
- Rationality and Rational Agents
- BDI Agents Model
- Autonomy in Agent
- PEAS Analysis
- Agents vs Other Software
- Agent Programs
- Types of Agents: Simple Reflex Agents, Model-Based Reflex Agents, Goal-Based Agents, and Utility-Based Agents
- Learning Agents
- How do the components of agent programs work?
- Overview of Agent Terminology
- Nature of Environments
- Properties of Task Environments
- Examples of Task Environments
- Knowledge and Knowledge with AI
- Knowledge Engineering
- Knowledge Acquisition
- Sources of Knowledge
- Inductive vs Deductive Reasoning
- Knowledge & Intelligence
- Knowledge Representation
- Levels of Knowledge & KR
- Knowledge Base & Management
- Knowledge-based Agent
- Database vs Knowledge-base
- Applications of Knowledge-base
- Types of Knowledge
- Cycle of KR in AI
- Knowledge Progression
- Knowledge (DIKW) Model
- Techniques of KR
- Approaches to KR in AI
- What is Prolog?
- The Structure of Prolog Program: Facts. Rules, Query
- Clause and Programs
- Some simple Prolog programs
- Syntax of Prolog Program
- Matching Operation
- Input and Output
- Meaning of Prolog Program
- How to write a rule?
- Types of Objects
- Operations on Lists
- Operators
- Expressions
- Predicates
- Looping
- Manu-base Program
- Prolog Programs on Tree and Graph Problems.
- Prolog Programs for DFA and NFA
- Prolog Programs for DFA and NFA
- Prolog Programs for Graph with cycle
- Programs with files
- Logic and Symbolic Logic
- Logic Types
- Propositional Logic (PL)
- Syntax, Semantics, and Terminology
- PL: Properties of Statements
- PL: Inference Rules
- Predicate Logic: Terminology
- First Order Predicate Logic (FOPL)
- Syntax and Semantics of FOPL
- WFFs and Properties of wffs
- Clausal Form and Resolution
- Conversion to Clausal Form
- Introduction to uncertainty
- Non-Monotonic vs Monotonic Reasoning
- Why we need more than first-order logic?
- Why do we need reasoning under uncertainty?
- Source of Uncertainty
- How to classify uncertainty?
- Making Decision: Uncertainty
Uncertainty - Analysis/Handling Techniques
- Fuzzy Logic
- Bayesian Probability Theory
- Hidden Markov Model (HMM)
- Artificial Neural Network (ANN)
- Problem solving
- Search and examples of search problems
- Searching for solutions
- Tree searches and search strategies
- Search algorithms
- Depth Limited Search Algorithm
- Iterative Deepening Depth First Search (IDDFS)
- Informed or Heuristic Search
- Best First Search Algorithm (Greedy search)
- Best First Search Algorithm (Greedy search)
- A* Search Algorithm
- Hill Climbing Algorithm
- What is an Expert System (ES)?
- Characteristics and Capabilities of ES
- Examples and Pioneer Works of ES
- Expert Systems vs Conventional Systems
- Components of Expert Systems
- Architecture of a typical Expert System
- Knowledge Base
- Inference Engine
- User Interface
- Explanation subsystem
- Blackboard
- Knowledge refinement subsystem
- Expertise and Expert
- Benefits and Limitations of Expert System
- Applications of Expert System